Expo & Prebuilt Quickstart
Overview
This guide will walk you through simple instructions to create a Video Conferencing app using the 100ms Prebuilt and Expo and test it using an Emulator or your Mobile Phone. Ensure that you are using the latest versions of the packages.
Create a sample expo app
This section contains instructions to create a simple Expo Video Conferencing app. We will help you with instructions to understand the project setup and complete code sample to implement this quickly.
Prerequisites
- A 100ms account if you don't have one already.
- Working Expo Local App Development Environment as we need to make changes in android and ios folders
- Familiar with basics of Expo.
- VS code or any other IDE / code editor
Create a Expo app
Once you have the prerequisites, follow the steps below to create a Expo app. This guide will use VS code but you can use any code editor or IDE.
-
Open your Terminal and navigate to directory/folder where you would like to create your app.
-
Run the below command to create Expo app:
npx create-expo-app my-expo-app && cd my-expo-app
- Install
expo-dev-client
lib, By installing this library, we will be able to install native libraries and modify project configuration
npx expo install expo-dev-client
- Test run your app
npx expo run:android
The above commands compile your project, using our locally installed Android SDK, into a debug build of your app. Learn more about running android app locally here
Install the Dependencies
After the Test run of your app is successful, you can install 100ms React Native Room Kit package in your app.
npx expo install @100mslive/react-native-room-kit
Install the dependencies of react-native-room-kit package
react-native-room-kit
package depends upon many other packages. These packages to be your app's direct dependencies so that react-native
can link them.
npx expo install @100mslive/react-native-hms \ @100mslive/types-prebuilt \ @react-native-community/blur \ @react-native-masked-view/masked-view \ @shopify/flash-list \ lottie-react-native \ react-native-gesture-handler \ react-native-linear-gradient \ react-native-modal \ react-native-reanimated \ react-native-safe-area-context \ react-native-simple-toast \ -- --legacy-peer-deps
Setup minimum deployment targets
We need to set minSdkVersion = 24
on Android platform and have to target minimum 13.0
iOS SDK. If you meet these requirements then you can skip this step, otherwise lets continue -
- Install
expo-build-properties
library, This library helps us to set minimum deployment targets using expo config
npx expo install expo-build-properties -- --save-dev --legacy-peer-deps
You can learn more about this library here
- Add below
expo-build-properties
plugin config in your expo plugin file that is root levelapp.json
file
[ "expo-build-properties", { "android": { "minSdkVersion": 24 }, "ios": { "deploymentTarget": "13.4" } } ]
Example app.json with above config plugin changes:
{ "expo": { "plugins": [ [ "expo-build-properties", { "android": { "minSdkVersion": 24 }, "ios": { "deploymentTarget": "13.4" } } ] ] } }
Setup Permissions for App
Since the App and @100mslive/react-native-room-kit package requires Camera & Microphone permissions, a package for requesting these permissions from users should also be installed.
- Install
expo-camera
library, It helps us to request Camera and Microphone permissions from user
npx expo install expo-camera -- --legacy-peer-deps
You can learn more about this library and its usage here
- Add below
expo-camera
plugin config in your expo plugin file that is root levelapp.json
file
[ "expo-camera", { "cameraPermission": "This Example app uses the camera for video calls", "microphonePermission": "This Example app uses the microphone for audio and video calls", "recordAudioAndroid": true } ]
Example app.json with above config plugin changes:
{ "expo": { "plugins": [ [ "expo-camera", { "cameraPermission": "This Example app uses the camera for video calls", "microphonePermission": "This Example app uses the microphone for audio and video calls", "recordAudioAndroid": true } ] ] } }
- Android platform requires some additional permissions, Add below permissions inside
android
inapp.json
config -
{ "expo": { ... "android": { ...
"permissions": ["android.permission.ACCESS_NETWORK_STATE","android.permission.CHANGE_NETWORK_STATE","android.permission.MODIFY_AUDIO_SETTINGS","android.permission.BLUETOOTH","android.permission.BLUETOOTH_CONNECT"]} ... } }
Configure Font Family
react-native-room-kit
package uses 'Inter' font family for texts be default. You need to setup 'Inter' fonts in your app.
- Install
expo-font
library, This library helps us to setup fonts for our app
npx expo install expo-font -- --legacy-peer-deps
You can learn more about this library and its usage here
-
Download Inter font family from Google Fonts.
-
Copy Paste downloaded Font Family variant files in
<root_of_project>/assets/fonts/
directory, It should look like -
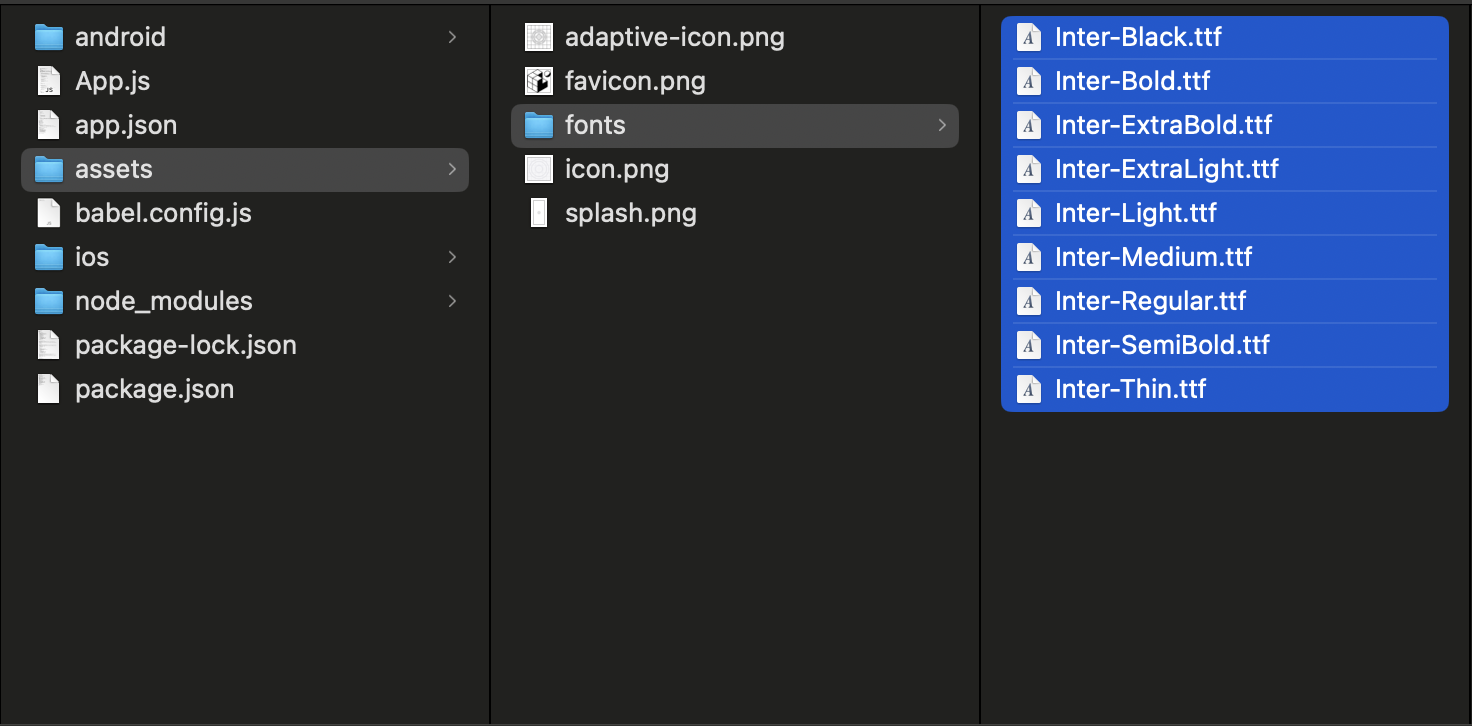
- Add below
expo-font
plugin config in your expo plugin file that is root levelapp.json
file
[ "expo-font", { "fonts": [ "./assets/fonts/Inter-Black.ttf", "./assets/fonts/Inter-Bold.ttf", "./assets/fonts/Inter-ExtraBold.ttf", "./assets/fonts/Inter-ExtraLight.ttf", "./assets/fonts/Inter-Light.ttf", "./assets/fonts/Inter-Medium.ttf", "./assets/fonts/Inter-Regular.ttf", "./assets/fonts/Inter-SemiBold.ttf", "./assets/fonts/Inter-Thin.ttf" ] } ]
Example app.json with above config plugin changes:
{ "expo": { "plugins": [ [ "expo-font", { "fonts": [ "./assets/fonts/Inter-Black.ttf", "./assets/fonts/Inter-Bold.ttf", "./assets/fonts/Inter-ExtraBold.ttf", "./assets/fonts/Inter-ExtraLight.ttf", "./assets/fonts/Inter-Light.ttf", "./assets/fonts/Inter-Medium.ttf", "./assets/fonts/Inter-Regular.ttf", "./assets/fonts/Inter-SemiBold.ttf", "./assets/fonts/Inter-Thin.ttf" ] } ] ] } }
Enable Background modes for iOS
You can enable background modes for audio in iOS. so that when you minimze the app, room audio can still be heared by the users.
Paste the following in your Info.plist
file -
<dict> ...
<key>UIBackgroundModes</key><array><string>audio</string></array>... </dict>
Additional Setup
You can follow ScreenShare setup and AudioShare setup guides to add ScreenShare and Audio Share features in your app.
After doing changes related to ScreenShare feature, To use screenshare feature on iOS, pass appGroup
and preferredExtension
options to HMSPrebuilt
componnets -
<HMSPrebuilt roomCode="..." options={{ ... ios: {
appGroup: "...";preferredExtension: "...";} }} />
Apply changes in native platform folders
We have done so many changes in our project, Run below command to apply these changes in native Android and iOS folders -
npx expo prebuild
Prebuilt Properties
<HMSPrebuilt roomCode={roomCode} // You can pass `roomCode` OR `token` prop, If you pass both `token` prop will be used token={authToken} // You can pass `roomCode` OR `token` prop, If you pass both `token` prop will be used options={{ userName: "John Appleseed", userId: "My_Unique_UserId", ios: { appGroup: 'group.rnroomkit', preferredExtension: 'live.100ms.reactnative.RNExampleBroadcastUpload', }, }} onLeave={handleRoomLeave} handleBackButton={isScreenFocused} autoEnterPipMode={true} /> const handleRoomLeave: OnLeaveHandler = useCallback((reason) => { console.log(':: Reason for Leaving the Room > ', reason); navigation.navigate('HomeScreen'); }, []);
Complete code example
Now that your project setup is complete, let's replace the code in the App.js
file with the complete code sample below -
import 'react-native-gesture-handler'; import React, { useEffect, useState } from 'react'; import { StatusBar } from 'expo-status-bar'; import { StyleSheet, Button, View } from 'react-native'; import { HMSPrebuilt } from '@100mslive/react-native-room-kit'; import { GestureHandlerRootView } from 'react-native-gesture-handler'; import { useFonts } from 'expo-font'; import { requestCameraPermissionsAsync, requestMicrophonePermissionsAsync } from 'expo-camera'; const App = () => { // LOAD REQUIRED FONTS const [fontsLoaded, fontError] = useFonts({ 'Inter-Bold': require("./assets/fonts/Inter-Bold.ttf"), 'Inter-Medium': require("./assets/fonts/Inter-Medium.ttf"), 'Inter-Regular': require("./assets/fonts/Inter-Regular.ttf"), 'Inter-SemiBold': require("./assets/fonts/Inter-SemiBold.ttf"), }); const [showHMSPrebuilt, setShowHMSPrebuilt] = useState(false); // Asking Camera & Microphone permissions from user useEffect(() => { Promise.allSettled([ requestCameraPermissionsAsync(), requestMicrophonePermissionsAsync(), ]) .then((results) => { console.log('Permissions Result: ', results); }) .catch((error) => { console.log('Permissions Error: ', error); }); }, []); // If fonts are not loaded then show nothing if (!fontsLoaded && !fontError) { return null; } // If an error occurred while loading fonts, show it if (!!fontError) { return <Text>{fontError.message}: {fontError.message}</Text> } // Content return ( <GestureHandlerRootView style={{ flex: 1 }}> <View style={styles.container}> <StatusBar barStyle={'dark-content'} /> {showHMSPrebuilt ? ( <HMSPrebuilt roomCode='rlk-lsml-aiy' options={{ userName: "John Appleseed" }} onLeave={() => setShowHMSPrebuilt(false)} /> ) : ( <View style={styles.joinContainer}> <Button title='Start' onPress={() => setShowHMSPrebuilt(true)} /> </View> )} </View> </GestureHandlerRootView> ); }; const styles = StyleSheet.create({ container: { flex: 1, }, joinContainer: { flex: 1, alignItems: 'center', justifyContent: 'center' } }); export default App;
Test the app
Follow the instructions in one of the tabs below based on the target platform you wish to test the app.
npx expo run:android
The above commands compile your project, using our locally installed Android SDK, into a debug build of your app. Learn more about running Android app locally here
Check Deployed Sample Apps
You can download and check out the 100ms React Native deployed apps -
🤖 Download the Sample Android App here
📲 Download the Sample iOS App here